7.3 Process Termination
Normal termination occurs in five ways:
- Return from main
- Calling
exit
- Calling
_exit
or_Exit
- Return of the last thread from its start routine
- Calling
pthread_exit
from the last thread
Abnormal termination occurs in three ways:
- Calling
abort
- Receipt of a signal
- Response of the last thread to a cancellation request
Exit Functions
#include <stdlib.h>
void exit(int status);
void _Exit(int status);
#include <unistd.h>
void _exit(int status);
_Exit
and_exit
return to kernel immediatelyexit
performs certain cleanup processing and then return to kernel (callfclose
to all open stream for example)
exit(0)
is the same as return 0
in the main
function.
When exit status undefined:
- Call exit function without an exit status
main
does a return without a return value- the
main
function is not declared to return an integer
If the return type of main
is an integer, both explicit return 0 or implicit return ("falls off the end") will make the exit status 0
ISO C 1999 implies that the return type of main defaults to int
.
main() {} // Return value is 0 in c99, undefined before c99
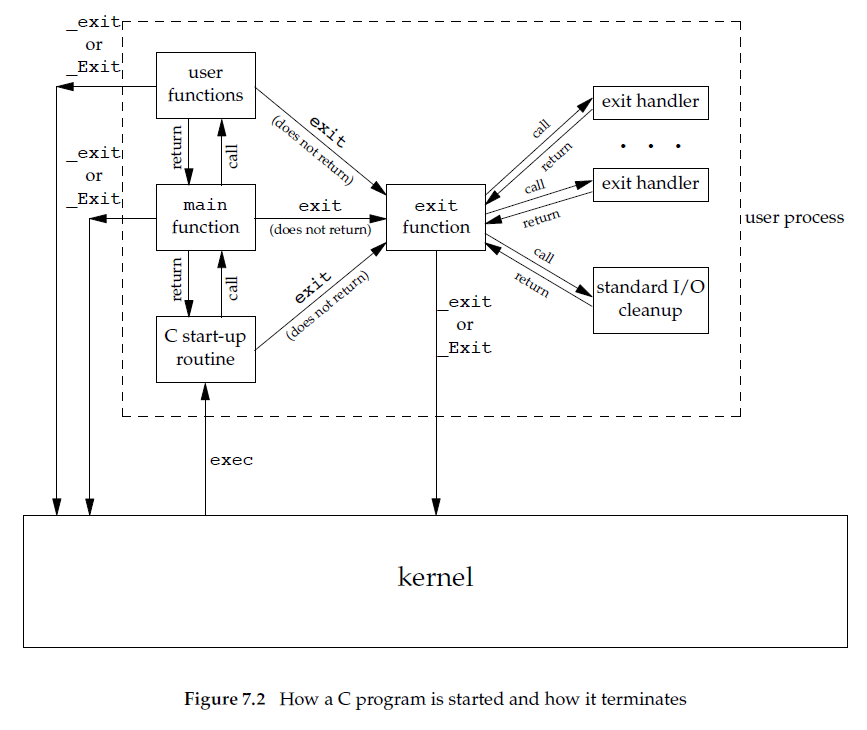
7.5 Environment List
extern char **environ;
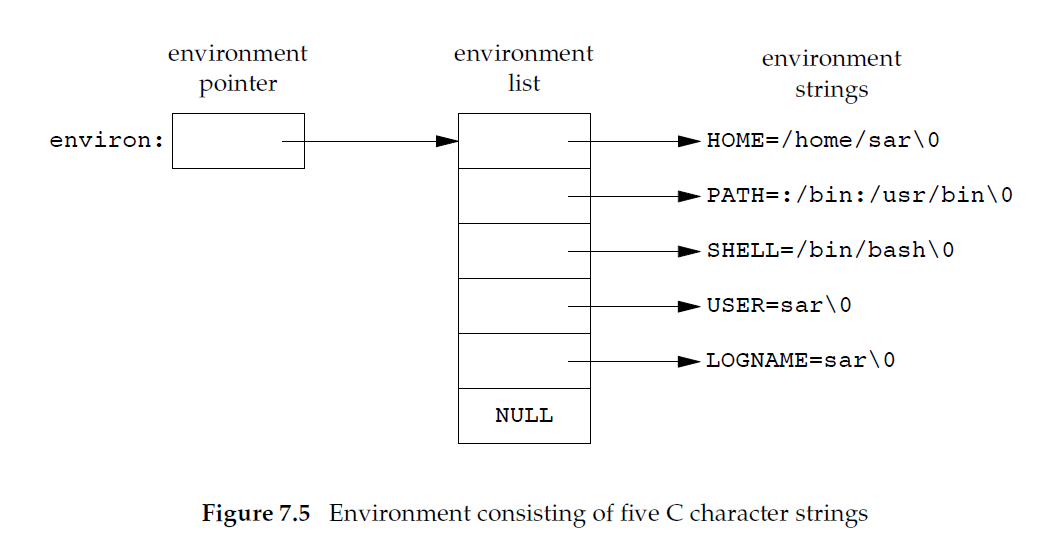
7.6 Memory Layout of a C program
This section simply describe the memory layout of a C program. Refer to CSAPP for more information.
7.8 Memory allocation
Although sbrk
can expand or contract the memory of a process, most versions of malloc
and free never decrease their memory size. The space that we free is available for a later allocation, but the freed space is not usually returned to the kernel; instead, that space is kept in the malloc
pool.
This may explain why the memory consumed by a process rarely decreases.
7.9 Environment Variables
Warning: don't use the putenv
function. putenv
is free to place the *name=value* string passed to it directly into the environment, without copy it. So it would be an error to pass a string allocated on the stack, since the memory would be reused after we return from the current function.
7.10 setjmp
and longjmp
Functions
Automatic, Register, and Volatile Variables
Refer to the code files setjmp_variables.c
.
When we return to the code as a result of the longjmp
, do the variables have values corresponding to those when the setjmp
was previous called (i.e. their values rolled back), or are their values left alone?
- Most implementations do not try to roll back automatic variables and register variables. (*seems not like this*)
- If you have an automatic variables that you don't want rolled back, define it with the
volatile
attribute. - Global or static variables are left alone when
longjmp
is executed.
Exercises
Q: 7.4 Some UNIX system implementations purposely arrange that, when a program is executed, location 0 in the data segment is not accessible. why?
A: This provides a way to terminate the process when it tries to dereference a null pointer, a common C programming error.